Overview
Models
aiXplain has an ever-expanding catalog of ready-to-use AI models by different suppliers (e.g. AWS, Microsoft, Google, Meta, etc.) for various functions (e.g. Machine Translation, Speech Recognition, Large Language Modeling, Sentiment Analysis, etc.). These models are available on-demand, can be connected together into Pipelines and Agents.
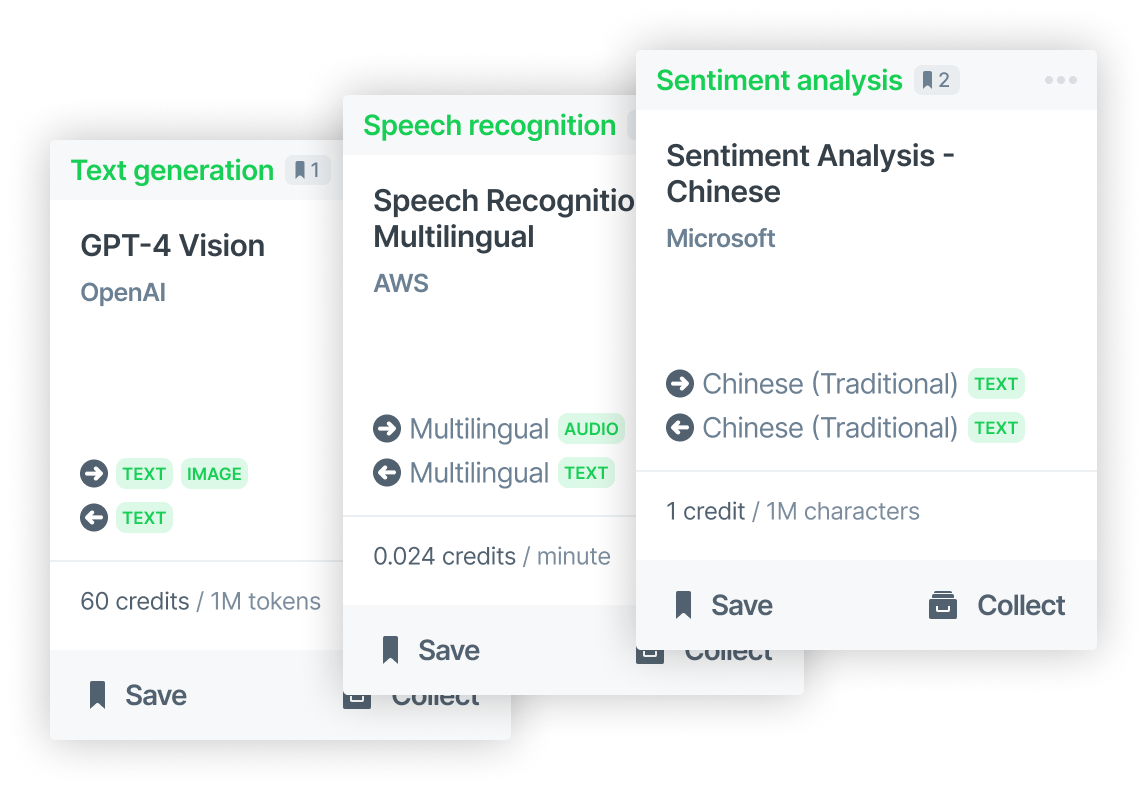
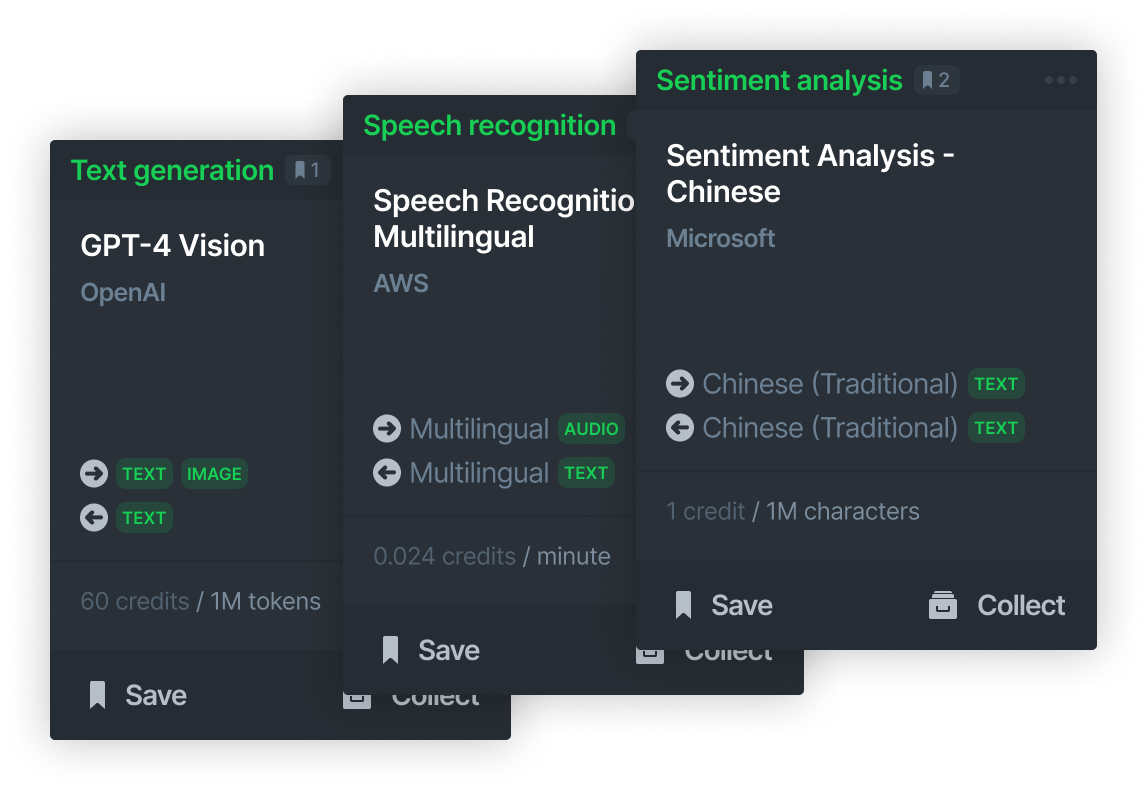
Curation
aiXplain's models are categorized using several filters, such as Function, Supplier and Modalities (e.g. source language, target language) to make searching easier.
Standardization
All aiXplain models can be run using the same syntax via the SDK, and our standardizations allow for model swapping in your pipelines.
Onboarding
You can onboard your own models onto aiXplain, making them accessible for deployment and utilization within your applications.
How to search the marketplace
This will walk you through the process of searching the marketplace for various aiXplain assets including models, metrics, and data.
- Models
- Metrics
- Data
Models
aiXplain's collection of models are searchable using queries, filters and are directly accessible using thier IDs (unique identifiers).
Models are searchable on the SDK using the following search parameters:
- Python
- Swift
Parameter | Type | Default | Description |
---|---|---|---|
query | Optional[Text] | "" | Search query to filter models based on their name or description. |
function | Optional[Function] | None | AI function filter (e.g., Translation, Text Generation). |
suppliers | Optional[Union[Supplier, List[Supplier]]] | None | Filter models by suppliers (e.g., AWS, Google, Microsoft). |
source_languages | Optional[Union[Language, List[Language]]] | None | Filter models based on their input language(s). |
target_languages | Optional[Union[Language, List[Language]]] | None | Filter models based on their output language(s). |
is_finetunable | Optional[bool] | None | Specify if models should support fine-tuning. |
ownership | Optional[Tuple[OwnershipType, List[OwnershipType]]] | None | Filter models by ownership type (e.g., SUBSCRIBED, OWNER). |
sort_by | Optional[SortBy] | None | Attribute to sort the retrieved models (e.g., name, creation date). |
sort_order | SortOrder | SortOrder.ASCENDING | Specify the sorting order (ascending or descending). |
page_number | int | 0 | Page number for paginated results. |
page_size | int | 20 | Number of results to retrieve per page. |
Parameter | Type | Default | Description |
---|---|---|---|
query | Optional[Text] | "" | Search query to filter models based on their name or description. |
function | Optional[Function] | None | AI function filter (e.g., Translation, Text Generation). |
- Python
- Swift
Let's use query
, function
, source_languages
, target_languages
and suppliers
to search for translation models from English to Canadian French.
from aixplain.factories import ModelFactory
from aixplain.enums import Function, Language, Supplier
model_list = ModelFactory.list(
"Canada",
function=Function.TRANSLATION,
source_languages=Language.English,
target_languages=Language.French,
suppliers=[Supplier.AWS, Supplier.GOOGLE, Supplier.MICROSOFT],
)["results"]
for model in model_list:
print(model.__dict__)
Use the _member_names_
attribute to see the list of available function types, languages and suppliers.
Function._member_names_
Language._member_names_
Supplier._member_names_
Let's use query
and function
to search for translation models from English to Canadian French.
let provider = ModelProvider()
Task {
let query = ModelQuery(query: "English to French Canada", functions: ["translation"])
let result = try? await provider.list(query)
result?.forEach { model in
dump(model)
}
}
The best way to view a list of all available functions is to check Discover. We will add a programmatic way to get this information to Swift in future. We will also soon display all models (and functions) in the Docs. 👍
Direct Access
Once you know a model's ID, you can access the model directly (without searching for it).
EXAMPLE
OpenAI's GPT-4 model has ID 6414bd3cd09663e9225130e8
.
Instantiate a model object
- Python
- Swift
from aixplain.factories import ModelFactory
model = ModelFactory.get('6414bd3cd09663e9225130e8')
model.__dict__
import AiXplainKit
let model = ModelProvider().get("6414bd3cd09663e9225130e8")
dump(model)
Once you have identified a suitable model, you can use it for inference, benchmarking, finetuning, or integrating it into custom AI pipelines.
Metrics
Metrics are searchable on the SDK using the following search parameters:
hypothesis
(Hypothesis): The metric's hypothesis modality. (Text, Audio, Image, Video, Label, Number.)is_source_required
(Source required): Should the metric use source. Defaults to None.is_reference_required
(Reference required):Should the metric use reference. Defaults to None.model_id
: ID of model for which metric is to be used. Defaults to None.
All parameters except query
can be passed as single items or lists.
View all available metrics by specifying no filters.
- Python
from aixplain.factories import MetricFactory
metric_list = MetricFactory.list(page_size=50)["results"]
for metric in metric_list:
print(metric.__dict__)
Let's search for all metrics compatible with GPT-4
that require a reference input.
- Python
metric_list = MetricFactory.list(
model_id=model.id,
is_reference_required=True,
)["results"]
for metric in metric_list:
print(metric.__dict__)
Direct Access
Once you know a metric's ID, you can access the metric directly (without searching for it).
EXAMPLE
BLEU has ID 639874ab506c987b1ae1acc6
.
Instantiate a metric object
- Python
from aixplain.factories import MetricFactory
bleu = MetricFactory.get('639874ab506c987b1ae1acc6')
bleu.__dict__
Once you have found your metric, you can use it for inference, finetuning and benchmarking.
Data
Data assets are searchable using queries and filters and directly accessible using their IDs (unique identifiers).
You cannot download the contents of a Dataset or Corpus.
Corpora
- Python
from aixplain.factories import CorpusFactory
corpus_list = CorpusFactory.list(
# query="",
# function=Function.TRANSLATION,
# language=[Language.English],
# data_type=DataType.TEXT,
# page_size=5
)["results"]
for corpus in corpus_list:
print(corpus.__dict__)
corpus = CorpusFactory.get("<CORPUS_ID>")
Datasets
- Python
from aixplain.factories import DatasetFactory
dataset_list = DatasetFactory.list(
# query="",
# function=Function.TRANSLATION,
# source_languages=[Language.English],
# target_languages=[Language.Spanish],
# data_type=DataType.TEXT,
# page_size=5
)["results"]
for dataset in dataset_list:
print(dataset.__dict__)
dataset = DatasetFactory.get("<DATASET_ID>")
Next, create or integrate these assets into AI agents to enhance your applications.
How to call an asset
The aiXplain SDK allows you to run models synchronously (Python) or asynchronously (Python and Swift). You can also process Data assets (Python).
Let's use Groq's Llama 70B as an example.
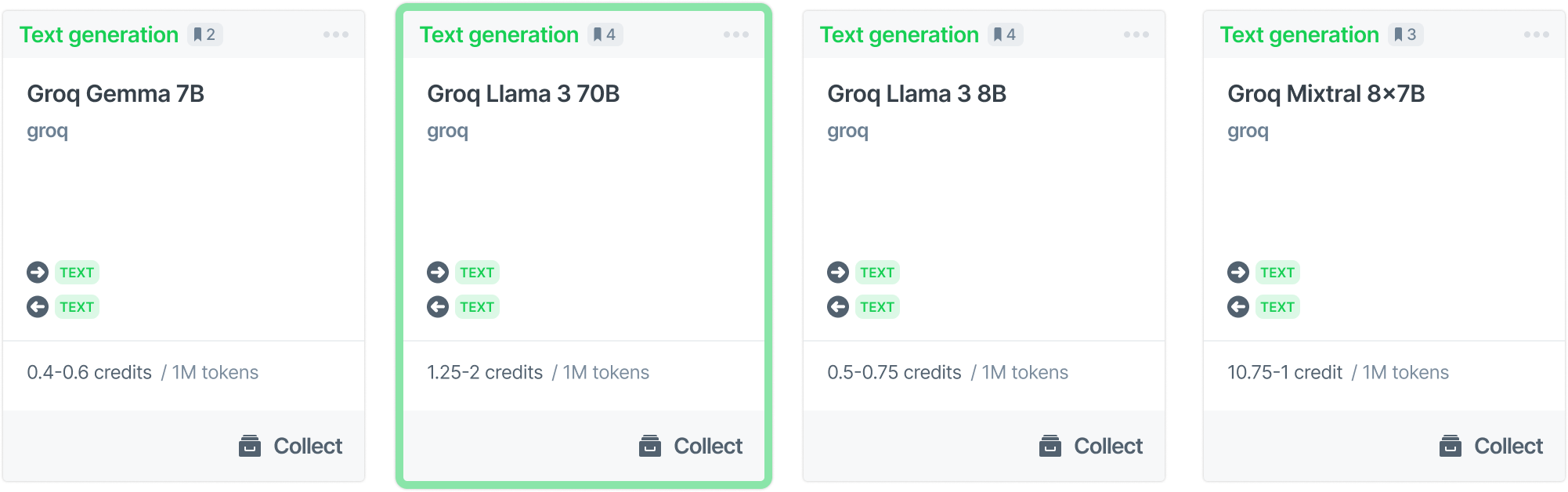
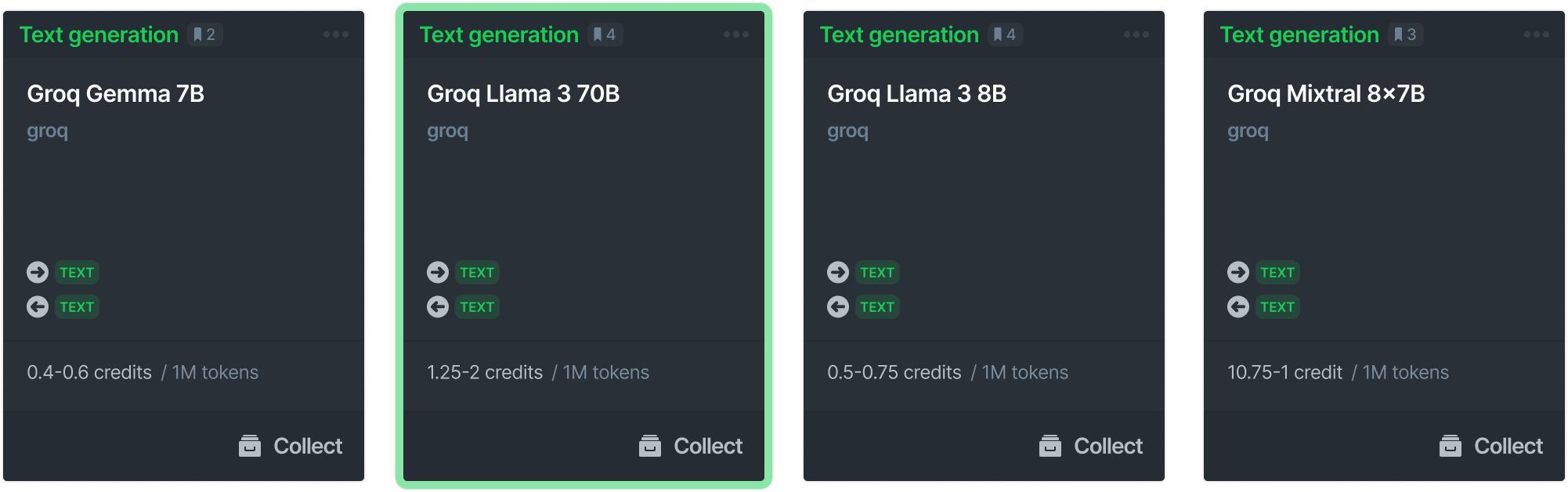
- Python
- Swift
from aixplain.factories import ModelFactory
from aixplain.enums import Supplier
model_list = ModelFactory.list(suppliers=Supplier.GROQ)["results"]
for model in model_list:
print(model.__dict__)
model = ModelFactory.get("6626a3a8c8f1d089790cf5a2")
let provider = ModelProvider()
Task {
let query = ModelQuery(query: "groq")
let result = try? await provider.list(query)
result?.forEach {
print($0.id, $0.name)
}
}
let model = ModelProvider().get("6626a3a8c8f1d089790cf5a2")
Model (and pipeline) inputs can be URLs, file paths, or direct text/labels (if applicable).
The examples below use only direct text.
Synchronous
- Python
model.run("Tell me a joke about dogs.")
Use a dictionary to specify additional parameters or if the model takes multiple inputs.
model.run(
{
"text": "Tell me a joke about dogs.",
"max_tokens": 10,
"temperature": 0.5,
}
)
Asynchronous
- Python
- Swift
start_response = model.run_async("Tell me a joke about dogs.")
start_response
Use the poll
method to monitor inference progress.
while True:
status = model.poll(start_response['url'])
print(status)
if status['status'] != 'IN_PROGRESS':
break
time.sleep(1) # wait for 1 second before checking again
let result = model.run("Tell me a joke about dogs.")
print(result)
Use a dictionary to specify additional parameters or if the model takes multiple inputs.
let result = try await model.run({
"text": "Tell me a joke about dogs.",
"max_tokens": 10,
"temperature": 0.5
})
print(result)
Process Data Assets
You can also perform inference on Data assets (Corpora or Datasets). You will need to onboard a data asset to use it.
Inference on Data assets is only available in Python.
Each data asset has an ID, and each column in that data asset has an ID, too. Specify both to perform inference:
- Python
With these steps, you can easily call models, pipelines, and integrate them into your agents to perform AI tasks.